Overview
This library is written in JavaScript and contains everything to add a clickable overlay or any content that you want to add on top of your "interactive video".To comunicate with the interactive video (i.e the engine), the content context uses a adways.content.HVBridge singleton named adways.hvBridgePool.
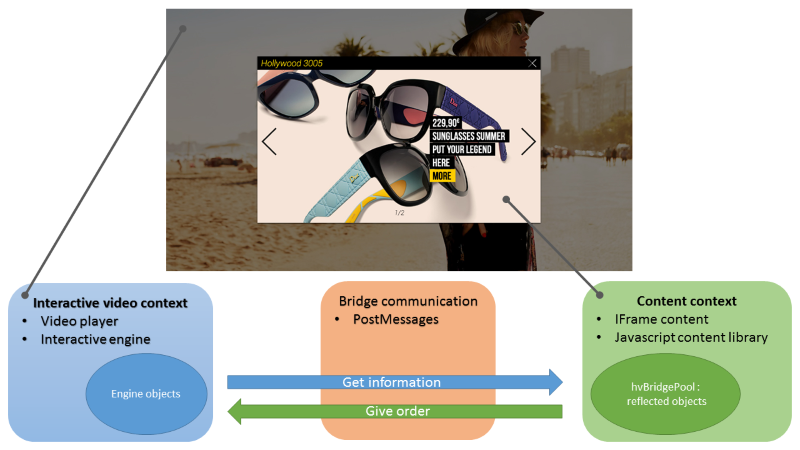
- the main video (play, pause, seek to, playhead time, etc.)
- the enrichments (open, close, etc.)
- the scene
Each of these objects has a "mirror" one inside the content that reflects its state and let you calling methods on it, in the same way as you generally call a method on an object. The mirror is reponsible of:
- correctly transmitting the order by automatically choosing the best way yo achieve this (with the "messaging" API as an exemple).
- bringing you its states (whether the current enrichment is opened or not, etc)
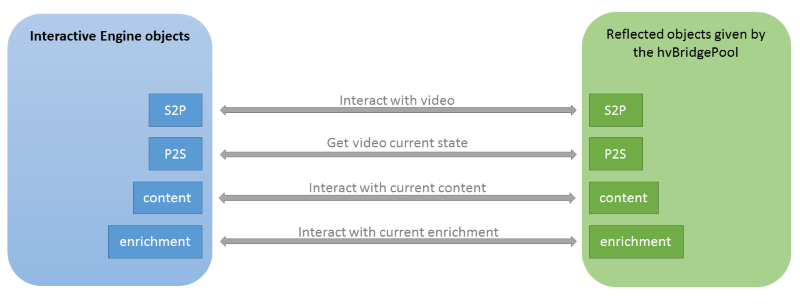
Example : How to instantiate the adways.content.HVBridge singleton and communicate with the interactive video engine
First of all, Adwayslib.js must be included
<script src="//d1xswutoby7io3.cloudfront.net/content/js/Adways-content/1.0.0/release.Adways-min.js"></script>
To start a communication between the engine (the interactive video) and the content, the bridge must be connected
/* * Check if adways.hvBridge is ready. * If adways.hvBridge is not ready add an adways.hv.bridge.events.READY event listener */ if (adways.hvBridge.isReady()) hvBridgeReadyCB(); else adways.hvBridge.addEventListener(adways.hv.bridge.events.READY, hvBridgeReadyCB);
Once the bridge is ready, the connection with the enrichement can be established
/* Get enrichment state and interact with it */ var enrichment = adways.hvBridge.getCurrentEnrichment(); /* * If enrichment is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var enrichmentConnectedCB = function () { console.log("enrichment connected"); /* Get enrichment current activation state (openned or closed) */ enrichment.getActivated().addEventListener(adways.type.evt.BOOLEAN, function(e){ /* Here, activation state can be accessed by the disptacher of the event : */ console.log("is activated (dispatcher method) : "+e.getDispatcher().valueOf()); /* or, directly by the enrichment getActivated method */ console.log("is activated (getActivated method) : "+enrichment.getActivated().valueOf()); }); /* method to close the enrichment */ enrichment.close(); }; if(enrichment.isConnected()) enrichmentConnectedCB(); else { enrichment.addEventListener(adways.hv.bridge.events.CONNECTED, enrichmentConnectedCB); enrichment.connect(); }
Connection with content can be established
/* Get content state and interact with it */ var content = adways.hvBridge.getCurrentContent(); /* * If content is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var contentConnectedCB = function () { console.log("content connected"); }; if(content.isConnected()) contentConnectedCB(); else { content.addEventListener(adways.hv.bridge.events.CONNECTED, contentConnectedCB); content.connect(); }
Connection with P2S SceneController can be established to get video state
/* Get video state */ var p2s = adways.hvBridge.getP2S(); /* * If p2s is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var p2sConnectedCB = function () { console.log("p2s connected"); /* get video current time */ p2s.addEventListener(adways.resource.events.CURRENT_TIME_CHANGED, function(e){ /* Here, current time can be accessed by the disptacher of the event : */ console.log("current time (dispatcher method) : "+e.getDispatcher().getCurrentTime().valueOf()); /* or, directly by the SceneController getCurrentTime method */ console.log("current time (getCurrentTime method) : "+p2s.getCurrentTime().valueOf()); }); /* get video current play state */ p2s.addEventListener(adways.resource.events.PLAY_STATE_CHANGED, function(e){ /* Here, playstate can be accessed by the disptacher of the event : */ console.log("play state (dispatcher method) : "+e.getDispatcher().getPlayState().valueOf()); /* or, directly by the SceneController getPlayState method */ console.log("play state (getPlayState method) : "+p2s.getPlayState().valueOf()); /* * Both return adways.resource.playStates.PLAYING when video is playing * and adways.resource.playStates.PAUSE when video is paused */ }); }; if(p2s.isConnected()) p2sConnectedCB(); else { p2s.addEventListener(adways.hv.bridge.events.CONNECTED, p2sConnectedCB); p2s.connect(); }
Connection with S2P SceneController can be established to interact with video
/* Interact with video */ var s2p = adways.hvBridge.getS2P(); /* * If s2p is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var s2pConnectedCB = function () { console.log("s2p connected"); /* seek to 10 seconds */ s2p.setCurrentTime(10, true); /* Play video */ s2p.play(true); /* Pause video */ s2p.pause(true); }; if(s2p.isConnected()) s2pConnectedCB(); else { s2p.addEventListener(adways.hv.bridge.events.CONNECTED, s2pConnectedCB); s2p.connect(); }
Here is the full sample demo
<script src="[PATH_TO_ADWAYSLIB.JS]"></script> <script> var hvBridgeReadyCB = function () { /* Get enrichment state and interact with it */ var enrichment = adways.hvBridge.getCurrentEnrichment(); /* * If enrichment is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var enrichmentConnectedCB = function () { console.log("enrichment connected"); /* Get enrichment current activation state (openned or closed) */ enrichment.getActivated().addEventListener(adways.type.evt.BOOLEAN, function(e){ /* Here, activation state can be accessed by the disptacher of the event : */ console.log("is activated (dispatcher method) : "+e.getDispatcher().valueOf()); /* or, directly by the enrichment getActivated method */ console.log("is activated (getActivated method) : "+enrichment.getActivated().valueOf()); }); /* method to close the enrichment */ enrichment.close(); }; if(enrichment.isConnected()) enrichmentConnectedCB(); else { enrichment.addEventListener(adways.hv.bridge.events.CONNECTED, enrichmentConnectedCB); enrichment.connect(); } /* Get content state and interact with it */ var content = adways.hvBridge.getCurrentContent(); /* * If content is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var contentConnectedCB = function () { console.log("content connected"); }; if(content.isConnected()) contentConnectedCB(); else { content.addEventListener(adways.hv.bridge.events.CONNECTED, contentConnectedCB); content.connect(); } /* Get video state */ var p2s = adways.hvBridge.getP2S(); /* * If p2s is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var p2sConnectedCB = function () { console.log("p2s connected"); /* get video current time */ p2s.addEventListener(adways.resource.events.CURRENT_TIME_CHANGED, function(e){ /* Here, current time can be accessed by the disptacher of the event : */ console.log("current time (dispatcher) : "+e.getDispatcher().getCurrentTime().valueOf()); /* or, directly by the SceneController getCurrentTime method */ console.log("current time (getCurrentTime) : "+p2s.getCurrentTime().valueOf()); }); /* get video current play state */ p2s.addEventListener(adways.resource.events.PLAY_STATE_CHANGED, function(e){ /* Here, playstate can be accessed by the disptacher of the event : */ console.log("play state (dispatcher method) : "+e.getDispatcher().getPlayState().valueOf()); /* or, directly by the SceneController getPlayState method */ console.log("play state (getPlayState method) : "+p2s.getPlayState().valueOf()); /* * Both return adways.resource.playStates.PLAYING when video is playing * and adways.resource.playStates.PAUSE when video is paused */ }); }; if(p2s.isConnected()) p2sConnectedCB(); else { p2s.addEventListener(adways.hv.bridge.events.CONNECTED, p2sConnectedCB); p2s.connect(); } /* Interact with video */ var s2p = adways.hvBridge.getS2P(); /* * If s2p is not connected then add an adways.hv.bridge.events.CONNECTED event listener * and connect it. */ var s2pConnectedCB = function () { console.log("s2p connected"); /* seek to 10 seconds */ s2p.setCurrentTime(10, true); /* Play video */ s2p.play(true); /* Pause video */ s2p.pause(true); }; if(s2p.isConnected()) s2pConnectedCB(); else { s2p.addEventListener(adways.hv.bridge.events.CONNECTED, s2pConnectedCB); s2p.connect(); } } /* * Check if adways.hvBridge is ready. * If adways.hvBridge is not ready add an adways.hv.bridge.events.READY event listener */ if (adways.hvBridge.isReady()) hvBridgeReadyCB(); else adways.hvBridge.addEventListener(adways.hv.bridge.events.READY, hvBridgeReadyCB); </script>