SUMMARY
Overview
This library is written in JavaScript and loadable on all the major desktop and mobile web browsers (??compatibility table ref??). It contains all the required functionalities to implements an "interactive video".It is divided into 2 major parts:
- the engine: made of a lot of concepts, classes and design patterns.
- a high level "business logic": a set of tools that makes its use really simple.
The top of the iceberg lives in the "interactive" namespace and is easily accessible through an easy to use object of type adways.interactive.Experience.
Exemple #1: quick and simple interactive video setup
First of all, the JavaScript library must be included
<script src="//dj5ag5n6bpdxo.cloudfront.net/adways/libs/interactive/loader.js"></script>
Then, an instance of the "Experience" class should be created. The library provides a
method that performs all the required functionalities to build it correctly (deeper explanations on the behind of the scene later).
The only data it needs are:
- a publication ID that refers to a publicated project into the adways dashboard.
- a container, which should be a <div> identifier or directly the DOMElement. The video player will be set into the space made by this div.
So, assuming that there is a <div id="adways-interactive-video"> somewhere in the DOM, creating an experience is very simple:
var experience = adways.interactive.createExperience({ "publication": "GSPfHsV",//publication id "container": "adways-interactive-video" //container id });
Remark:
"the method
createExperience
is a tool, a "helper" that executes all the required commands for you, but you can
do it by yourself and then customize the final experience. Check the
Exemple #4: instanciating and filling the experience "manually"."
Now it is time to load every thing, just calling the experience's "load" method:
experience.load();
See the "Experience" class to know more about the other ways to create an experience such as adding the interactivity to an already instanciated player, or how to add listeners to monitor what the viewer is doing...
Mechanism
basis
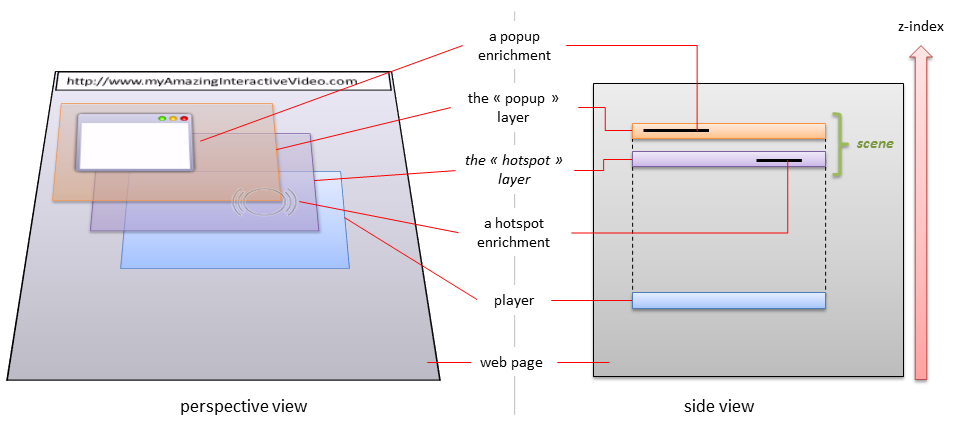
The Adways interactive engine adds multiple layers (the purple and orange plane) on top of a video player (the blue plane).
Those layers contain enrichments.
These enrichments are those elements that the engine add in front of the video, displaying additional information to the spectator and also to capture its interactions. Whether enrichment is a "hotspot" or a "popup", it is only a "functional" role choice, not 2 real different kinds of object. Hotspot and popup are the same elements, it is up to their configuration (attributes, behavior and layer they are added to) to make them acting as a hotspot or a popup. Moreover, further down the documentation, you will see that additional layers are available, but we will see that later in order to keep this introduction simple.
Some words about the layers:
- they are enrichments containers
- they are enrichments "floor" (z-index base)
- they are enrichments coordinates reference system
- they are graphically invisibles
- they are non "opaque": they do not catch user interactions so that a "mouse click" or a "finger touch" is still captured by the player so that the default player behavior is unchanged
Although a huge effort has been done to place those layers "at the same place" regardless the used player, some differences may appear: some players let the engine places layers behind the "controller" (play/pause button, seek bar, volume slider, etc.) and some do not. That impacts the visual result.
Enrichments
Coming soon.Scene
All animations and interactivities played in front of a video are held in a "Scene" object. It is the general interactivity conductor by transmitting:- the tempo from the player to all the time based elements (animations).
- information on the player and stream geometry to all the displayed elements.
To be completed.
Player and engine linkage
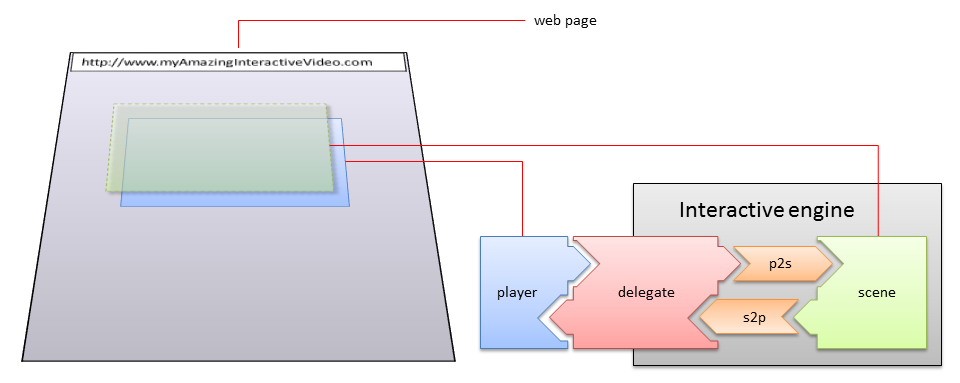
Remarks:
- the "interactive engine" is an abstract concept made of a lot of different concret elements.
- the default behavior of an "experience" is to setup and to free all these elements automatically; but it can be declutched (see the "Experience" class).
player
The player API entry point: the object that owns methods like "play", "pause", "seekTo", "getPlayheadTime", etc.
Since such APIs may change from a player to another, there is an intermediate object aka, the "delegate", reponsible of
establishing a clear communication channel between the player and the engine.
delegate
This object enables players interoperability. It knows both the player API and the interactive engine one. It has two major responsibilities depending on (1) the player or (2) the engine side::
- the player side, it is responsible of exchanging with the engine:
- giving information on the player state.
As an example, whether the player is paused or not, the current playhead time, the current volume, etc. - translating the engine's orders to intelligibles messages for the player, ie: calling the right player API method.
As an example, one player API may have aplay()
method whereas another one may have asetPlayState("play")
. This is up to the delegate to perform the right call.
- giving information on the player state.
- the engine side, it is responsible of managing the player. The engine does not have to know the exact player API, it count on the delegate capabilities to correctly translate its orders to the player.
That is why it is called a "delegate": whatever the considered side is, the communication is "delegated" to this object.
So it knows both APIs: the player one and the engine one. But to make it easier to implement, a "delegate developer" does not have to
investigate into the full engine API to understand how it works and what he can do with. Dealing with a player API on one hand, and with
the full Adways API engine on the other hand, might create confusionay. So on the engine side, there is only one class to know:
"SceneControllerWrapper"
It is instantiated 2 times for directional communication between the engine and the delegate
(see "s2p" and "p2s" objects).
"s2p" and "p2s" objects
These 2 objects establish a bi-directional communication pipe between the scene and the player delegate:
- the "p2s" object stands for the "from player to scene" channel. Used to report the player states to the scene (volume current playhead time, stream size, play state, etc.).
- the "s2p" object stands for the "from scene to player" channel. Used by the scene to give order to the player (pause the playback, change the playback rate, mute the sound, etc.).